In this getting started chapter we will look a demo to illustrate basic usage of Oracle Coherence when using it with Spring. This demo provides an example of using Coherence Spring’s Cache Abstraction.
The demo application is basically a super-simple event manager. We can create Events
and assign People
to them using
an exposed REST API. The data is saved in an embedded HSQL database. The caching is implemented at
the service layer.
When an Event
is created, it is not only persisted to the database but also put to the Coherence Cache.
Therefore, whenever an Event
is retrieved, it will be returned from the Coherence Cache. You can also delete
Events
, in which case the Event
will be evicted from the cache. You can perform the same
CRUD operations for people as well.
1. How to Run the Demo
In order to get started, please checkout the code from the coherence-community/coherence-spring[Coherence Spring Repository] GitHub repository.
$ git clone https://github.com/coherence-community/coherence-spring.git
$ cd coherence-spring
You now have checked out all the code for Coherence Spring. The relevant demo code for this Quickstart demo is under
samples/coherence-spring-demo/
.
There you will find 3 Maven sub-modules:
-
coherence-spring-demo-classic
-
coherence-spring-demo-boot
-
coherence-spring-demo-core
The first two Maven modules are essentially variations of the same app. The third module contains shared code.
coherence-spring-demo-classic |
Provides a demo using Spring Framework without Spring Boot |
coherence-spring-demo-boot |
Provides a demo using Spring Boot |
coherence-spring-demo-core |
Contains common code shared between the two apps |
In this chapter we will focus on the Spring Boot version. Since we checked out the project, let’s build it using Maven:
$ ./mvnw clean package -pl samples/coherence-spring-demo/coherence-spring-demo-boot
Now we are ready to run the application:
$ java -jar samples/coherence-spring-demo/coherence-spring-demo-boot/target/coherence-spring-demo-boot-4.1.0.jar
2. Interacting with the Cache
Once the application is started, the embedded database is empty. Let’s create an event with 2 people added to them using curl:
curl --request POST 'http://localhost:8080/api/events?title=First%20Event&date=2020-11-30'
This call will create and persist an Event
to the database. However, there is more going on. The created Event
is also
added to the Coherence Cache. The magic is happening in the Service layer, specifically in
DefaultEventService#createAndStoreEvent()
, which is annotated with @CachePut(cacheNames="events", key="#result.id")
.
The cacheNames
attribute of the @CachePut
annotation indicates the name of the underlying cache to use. As caches are
basically just a Map, we also need a key. In this case we use the expression #result.id
to retrieve the primary key of
the Event
as it was persisted. Thus, the saved Event
is added to the cache named events
and ultimately also
returned and printed to the console:
{
"id" : 1,
"title" : "First Event",
"date" : "2020-11-30T00:00:00.000+00:00"
}
We see that an Event with the id 1
was successfully created. Let’s verify that the cache put worked by inspecting
the cache using the open-source tool VisualVM.
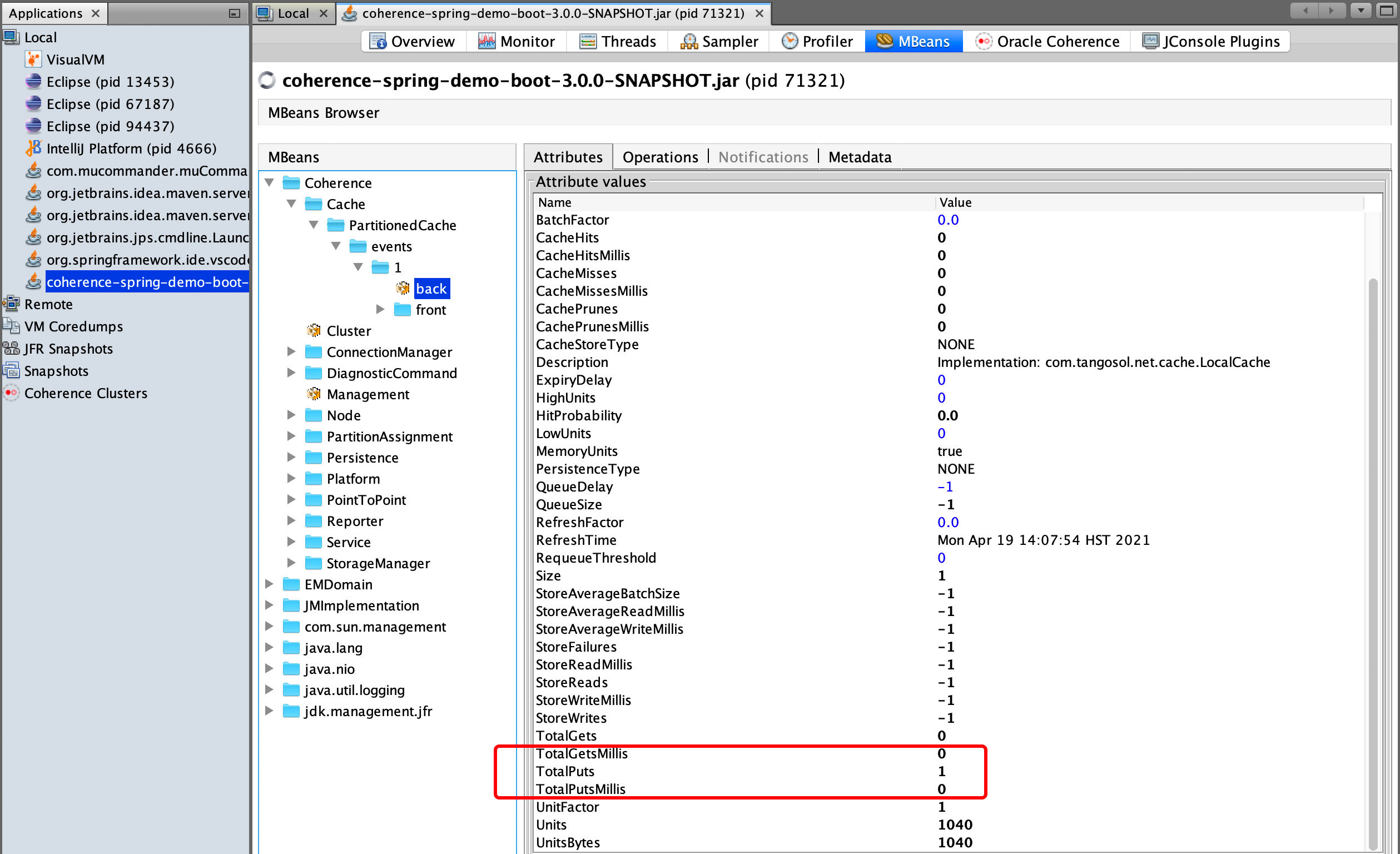
Under the MBeans
tab you will find the Cache MBeans, including and entry for the events
cache,
providing numerous statistical information regarding the cache.
$ curl --request GET 'http://localhost:8080/api/statistics/events'
You should see an entry for TotalPuts
of 1
.
When using VisualVM consider installing the respective Coherence VisualVM Plugin as it provides some additional insights and visualizations. |
Next, lets retrieve the Event using id 1:
curl --request GET 'http://localhost:8080/api/events/1'
The Event is returned. Did you notice? No SQL queries were executed as the value was directly retrieved from the Cache. Let’s check the statistics again, this time via the Coherence VisualVM Plugin:
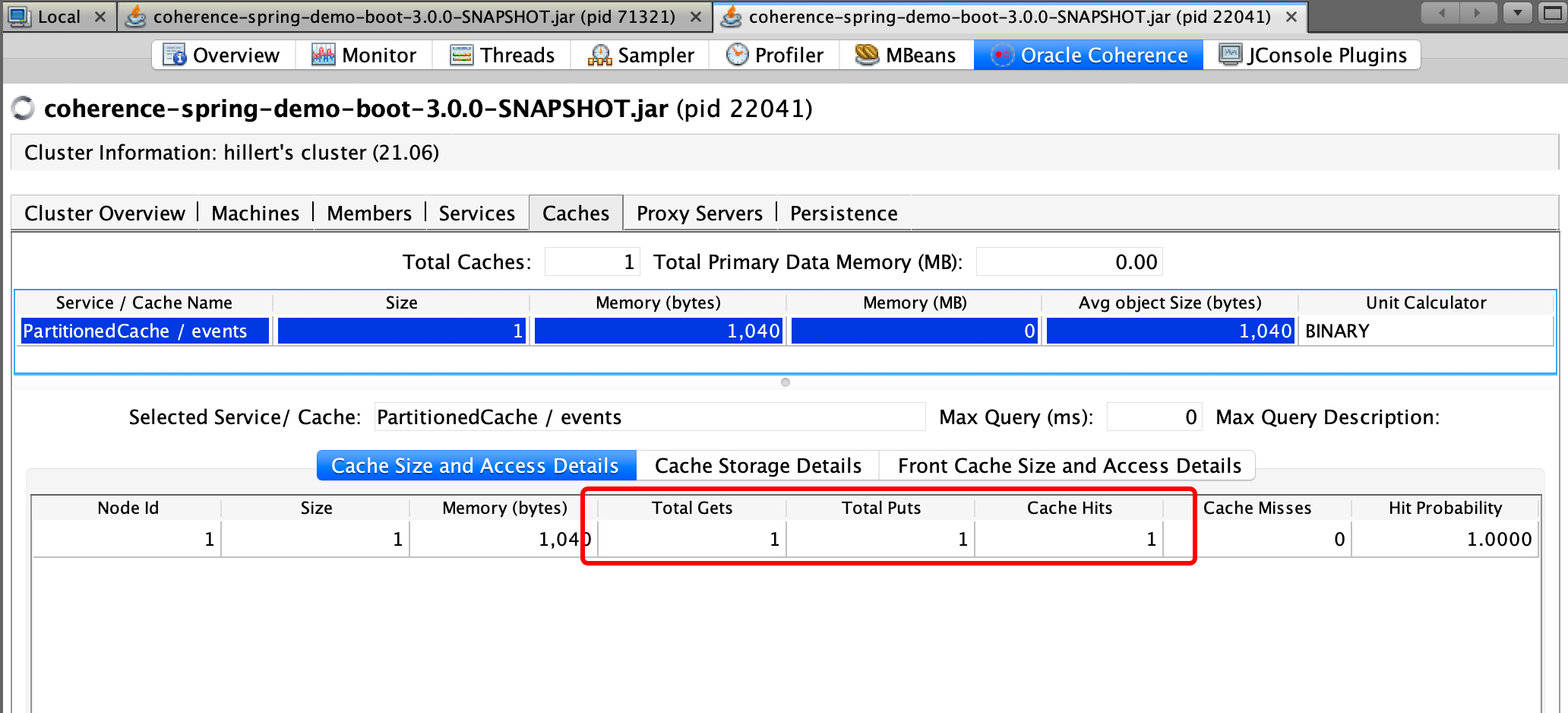
We will see now how values are being returned from the cache by seeing increasing cacheHits, e.g., "cacheHits" : 1
.
Let’s evict our Event with id 1 from the cache named events:
curl --request DELETE 'http://localhost:8080/api/events/1'
If you now retrieve the event again using:
curl --request GET 'http://localhost:8080/api/events/1'
you will see an SQL query executed in the console, re-populating the cache. Feel free to play along with the Rest API. We can, for example, add people:
curl --request POST 'http://localhost:8080/api/people?firstName=Conrad&lastName=Zuse&age=85'
curl --request POST 'http://localhost:8080/api/people?firstName=Alan&lastName=Turing&age=41'
curl --request GET 'http://localhost:8080/api/people'
Or assign people to events:
curl --request POST 'http://localhost:8080/api/people/2/add-to-event/1'
curl --request POST 'http://localhost:8080/api/people/3/add-to-event/1'
3. Behind the Scenes
What is involved to make this all work? Using Spring Boot, the setup is incredibly simple. We take advantage of Spring Boot’s AutoConfiguration capabilities, and the sensible defaults provided by Coherence Spring.
In order to activate AutoConfiguration for Coherence Spring you need to add the coherence-spring-boot-starter
dependency as well as the desired dependency for Coherence.
<dependency>
<groupId>com.oracle.coherence.spring</groupId>
<artifactId>coherence-spring-boot-starter</artifactId> (1)
<version>4.1.0</version>
</dependency>
<dependency>
<groupId>com.oracle.coherence.ce</groupId>
<artifactId>coherence</artifactId> (2)
<version>23.09.1</version>
</dependency>
1 | Activate Autoconfiguration by adding the coherence-spring-boot-starter dependency |
2 | Add the desired version of Coherence (CE or Commercial) |
In this quickstart example we are using Spring’s Caching abstraction and therefore, we
use the spring-boot-starter-cache
dependency as well:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
For caching you also must activate caching using the @EnableCaching
annotation.
@SpringBootApplication
@EnableCaching (1)
public class CoherenceSpringBootDemoApplication {
public static void main(String[] args) {
SpringApplication.run(CoherenceSpringBootDemoApplication.class, args);
}
}
1 | Activate the Spring Cache Abstraction |
Please see the relevant chapter on Caching in the Spring Boot reference guide.
With @EnableCaching
in place, Coherence’s autoconfiguration will also provide a CoherenceCacheManager
bean to the
application context.